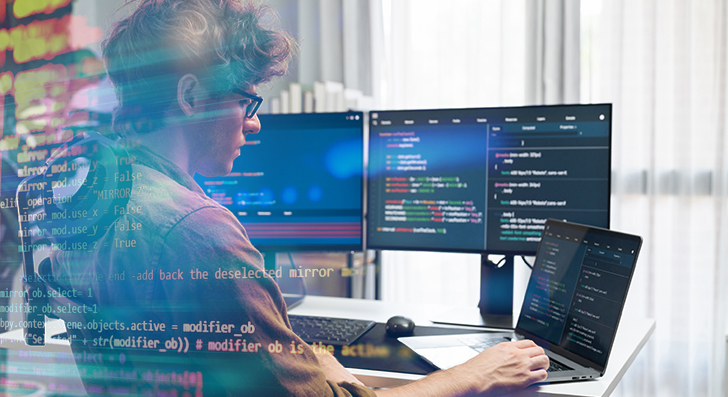
Scalability suggests your software can cope with progress—a lot more users, extra facts, and a lot more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety afterwards. Below’s a clear and realistic guidebook that will help you get started by Gustavo Woltmann.
Layout for Scalability from the Start
Scalability just isn't a little something you bolt on later on—it ought to be element of your prepare from the start. A lot of applications fall short after they mature quickly because the initial structure can’t manage the additional load. As being a developer, you'll want to Believe early regarding how your program will behave stressed.
Start by planning your architecture to generally be versatile. Prevent monolithic codebases where almost everything is tightly connected. Alternatively, use modular design or microservices. These patterns split your app into scaled-down, unbiased components. Every single module or company can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from working day 1. Will it want to manage a million consumers or maybe 100? Pick the correct kind—relational or NoSQL—depending on how your knowledge will mature. Plan for sharding, indexing, and backups early, even if you don’t require them but.
A different significant issue is to prevent hardcoding assumptions. Don’t compose code that only is effective under present situations. Think of what would materialize if your user base doubled tomorrow. Would your app crash? Would the database decelerate?
Use style patterns that assistance scaling, like concept queues or event-driven systems. These help your app handle far more requests with no receiving overloaded.
If you Create with scalability in mind, you're not just making ready for fulfillment—you happen to be cutting down long run problems. A well-prepared process is simpler to maintain, adapt, and grow. It’s improved to get ready early than to rebuild later on.
Use the proper Database
Choosing the suitable database is really a vital Component of constructing scalable applications. Not all databases are crafted the exact same, and using the Mistaken one can gradual you down as well as trigger failures as your application grows.
Start off by comprehending your details. Could it be extremely structured, like rows inside a desk? If Sure, a relational databases like PostgreSQL or MySQL is an efficient match. These are solid with relationships, transactions, and regularity. They also guidance scaling approaches like go through replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your knowledge is more versatile—like person activity logs, product catalogs, or paperwork—consider a NoSQL selection like MongoDB, Cassandra, or DynamoDB. NoSQL databases are improved at dealing with substantial volumes of unstructured or semi-structured information and might scale horizontally more simply.
Also, take into consideration your go through and write designs. Are you presently performing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases that will cope with high create throughput, as well as party-based information storage programs like Apache Kafka (for momentary details streams).
It’s also intelligent to Assume in advance. You might not require Superior scaling capabilities now, but deciding on a databases that supports them means you won’t require to switch later on.
Use indexing to hurry up queries. Prevent avoidable joins. Normalize or denormalize your data based on your access patterns. And usually check database efficiency while you expand.
To put it briefly, the ideal databases relies on your application’s composition, pace demands, And just how you assume it to increase. Just take time to choose correctly—it’ll preserve a great deal of problems later.
Optimize Code and Queries
Fast code is essential to scalability. As your application grows, every single tiny delay provides up. Improperly written code or unoptimized queries can decelerate effectiveness and overload your technique. That’s why it’s crucial that you Construct efficient logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away nearly anything unneeded. Don’t choose the most elaborate Option if an easy one is effective. Maintain your functions small, targeted, and straightforward to check. Use profiling equipment to locate bottlenecks—sites the place your code requires much too prolonged to run or works by using a lot of memory.
Future, have a look at your database queries. These normally sluggish things down a lot more than the code itself. Be sure Every question only asks for the data you really have to have. Keep away from SELECT *, which fetches almost everything, and as an alternative find certain fields. Use indexes to hurry up lookups. And steer clear of undertaking a lot of joins, Specifically throughout large tables.
In case you see the identical facts being asked for again and again, use caching. Retailer the effects temporarily making use of instruments like Redis or Memcached so you don’t must repeat high priced functions.
Also, batch your databases operations once you can. In place of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Remember to examination with substantial datasets. Code and queries that do the job fine with 100 information may well crash if they have to handle 1 million.
In brief, scalable apps are quickly apps. Maintain your code restricted, your queries lean, and use caching when essential. These techniques assistance your software continue to be sleek and responsive, at the same time as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to handle much more consumers and even more targeted traffic. If almost everything goes by way of one particular server, it can promptly turn into a bottleneck. That’s in which load balancing and caching are available in. Both of these instruments enable maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of just one server undertaking each of the perform, the load balancer routes customers to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this straightforward to put in place.
Caching is about storing information quickly so it might be reused promptly. When consumers ask for the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to provide it from your cache.
There are two popular forms of caching:
1. Server-aspect caching (like Redis or Memcached) stores facts in memory for quick obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near to the person.
Caching decreases databases load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t transform frequently. And generally make certain your cache is up-to-date when data does modify.
To put it briefly, load balancing and caching are straightforward but highly effective equipment. Alongside one another, they help your app tackle much more end users, continue to be quickly, and Get well from complications. If you plan to increase, you would like each.
Use Cloud and Container Instruments
To make scalable applications, you require tools that let your app increase conveniently. That’s where cloud platforms and containers are available in. They provide you overall flexibility, lower set up time, and make scaling much smoother.
Cloud platforms like Amazon Internet Companies (AWS), Google Cloud System (GCP), and Microsoft Azure Enable you to hire servers and products and services as you will need them. You don’t really need to obtain components or guess long run potential. When targeted visitors increases, you are able to include much more sources with only a few clicks or instantly making use of automobile-scaling. When site visitors drops, it is possible to scale down to save cash.
These platforms also present expert services like managed databases, storage, load balancing, and stability instruments. You may center on making your app as opposed to handling infrastructure.
Containers are An additional important tool. A container offers your application and almost everything it has to run—code, libraries, configurations—into just one unit. This causes it to be straightforward to move your application involving environments, out of your laptop to the cloud, without the need of surprises. Docker is the most well-liked Instrument for this.
When your application makes use of numerous containers, tools like Kubernetes make it easier to deal with them. Kubernetes handles here deployment, scaling, and recovery. If a person portion of your respective app crashes, it restarts it automatically.
Containers also help it become simple to different portions of your app into expert services. You'll be able to update or scale parts independently, and that is great for general performance and dependability.
In short, employing cloud and container resources suggests you'll be able to scale speedy, deploy conveniently, and Recuperate promptly when difficulties materialize. If you'd like your application to develop without the need of limitations, start out utilizing these instruments early. They save time, lessen hazard, and enable you to continue to be focused on constructing, not correcting.
Keep track of Almost everything
Should you don’t watch your application, you won’t know when factors go Completely wrong. Monitoring will help the thing is how your application is carrying out, place difficulties early, and make better choices as your app grows. It’s a critical part of creating scalable programs.
Get started by tracking fundamental metrics like CPU utilization, memory, disk House, and response time. These tell you how your servers and providers are executing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application much too. Regulate how long it takes for customers to load webpages, how often mistakes take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s happening inside your code.
Set up alerts for important issues. For instance, In case your response time goes above a Restrict or simply a company goes down, you'll want to get notified straight away. This can help you deal with difficulties rapidly, typically just before consumers even discover.
Monitoring is usually handy any time you make alterations. When you deploy a whole new characteristic and see a spike in faults or slowdowns, you may roll it back again prior to it results in authentic hurt.
As your app grows, targeted visitors and facts boost. With out checking, you’ll overlook signs of issues until finally it’s as well late. But with the ideal equipment in place, you keep in control.
To put it briefly, monitoring assists you keep the app reliable and scalable. It’s not almost spotting failures—it’s about knowledge your method and ensuring that it works perfectly, even under pressure.
Remaining Ideas
Scalability isn’t only for large providers. Even tiny applications require a robust foundation. By planning cautiously, optimizing correctly, and utilizing the proper applications, you'll be able to Establish apps that mature easily without the need of breaking under pressure. Start off small, Feel major, and Develop sensible.